This post shows how to create a dot moving along a path using SVG and JavaScript (ES2015). In this case it’s a dot, but of course it could be anything.
Create graphics with Inkscape
Let’s create an image consisting of a curve and an object that we want to move along that curve. I’m using Inkscape as SVG editor, but you can use Adobe Illustrator as well, or even create the SVG file manually.
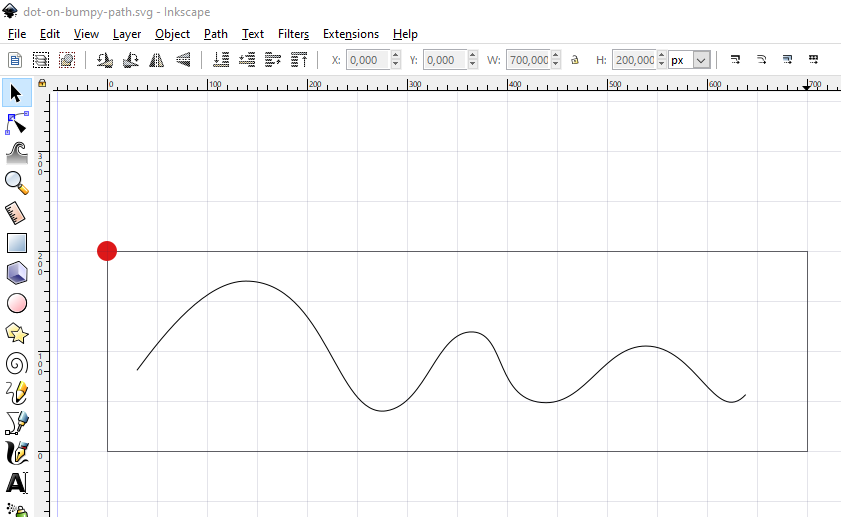
The image shows the two elements we need. The curly curve is given the id curve, the dot has id dot. Note the position of the dot: it’s placed on the origin.
The (cleaned-up) code for that graphic would be something like this:
Beware of group transforms
For more complicated graphics you will likely group elements or separate them in layers (which are actually groups too). In that case make sure you don’t have a transform applied to the group, to avoid problems addressed below. A simple trick to do this, is to ungroup and regroup the group. This transfers the transform data to the geometric elements inside the group.
Add graphics to HTML page
Simply paste the SVG element in the body of the HTML document, or, if using PHP, include the SVG file.
Put the dot on the spot
If we want to move the dot along the curve, obviously we first have to find out how to position the dot on a particular spot. To do this we’ll use two methods for SVG geometry: getTotalLength and getPointAtLength.
getTotalLength measures the total length of the path, while getPointAtLength gives the position on the path given the distance from the starting point. More on SVGGeometryElements.
Let’s use a parameter u running from 0 to 1. When u = 0, the dot is on the starting point, while u = 1 corresponds with the end point. To put the dot half way we could write this:
The following image shows the result in the browser window.
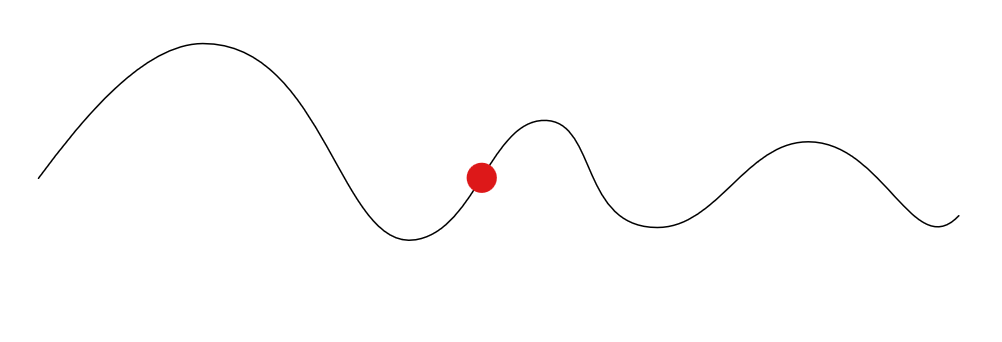
Group transform issue
Suppose we organized our SVG file and added the curve in a group or layer. In that case getPointAtLength would not take any transforms applied to that group into account, so translating the dot based on the outcome of getPointAtLength would probably put the dot on the wrong place. Therefor we warned above to avoid transforms on groups or layers in the SVG document.
Move it
Let’s encapsulate the code for dot in an object, so we can repeatedly move the dot, without making a mess of the code.
To control the animation, let’s create another object, anim, that can be started specifying the duration of the animation. This demo restarts the animation a second after it’s finished.
In the function run (line 10 in the code above) we calculated the progress parameter u, by dividing the time the animation is running by the total running time. This expresses the progress of the animation on a scale from 0 to 1. If we are beyond the time set as duration we finish the animation by limiting u to 1.
When the DOM is ready, just intitialise the dot, and start the animation:
Repo
Source and transpiled code at github.
Add timing function
The follow-up post Ease a dot along a curve demonstrates the effect of easing (or timing) functions to an animation.
Pause, resume
There’s also a variant available on Codesandbox that lets you pause and resume the animation on mouseover
and mouseleave
.