This post offers solutions for
- Intersection of a parabola with a line: find the incidence point
- Find the Bezier control point to draw section of a given parabola
- Solve quadratic equation
Introduction
This story begins with my attempt to simulate an object bouncing around inside a box of a certain shape (a polygon). I thought that maybe this would produce some cool graphics somehow, or the bouncing object would behave in unexpected and interesting ways.
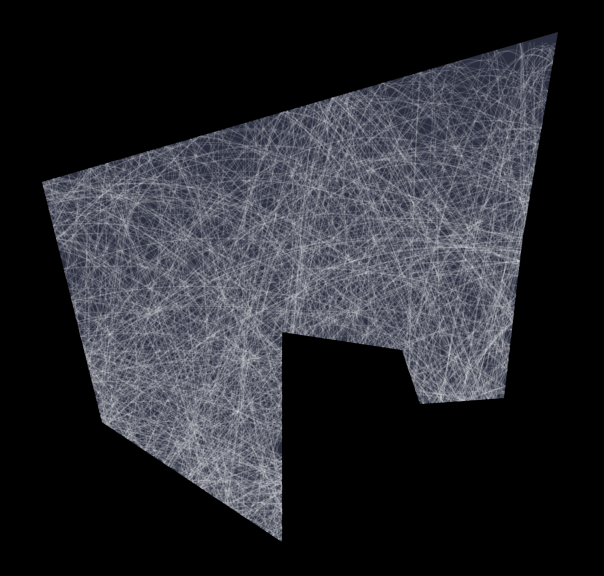
The following image shows the simulation after a few steps. The bounce is the result of a gravitational force exerted on the object.
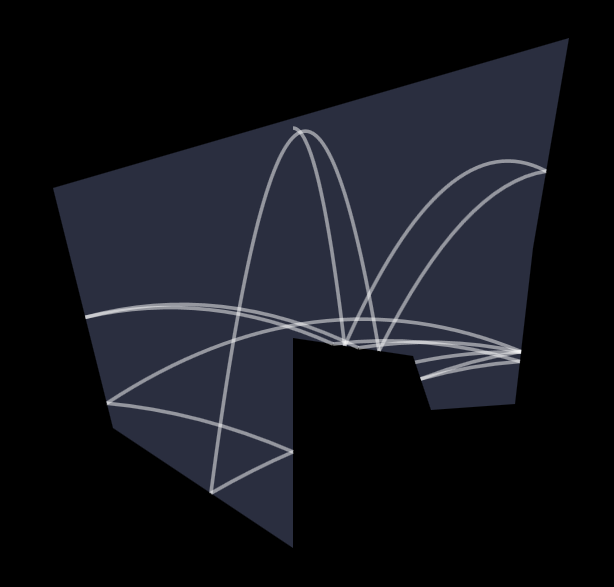
To draw this it’s necessary to find out the position of the next bounce. Also, the position where the object would hit a polygon edge.
The bouncing point describes parabolas, while the edges of the polygon are line segments. So the problem is: Find the point where a given parabola intersects a given line.
Parametric representations
Consider a parabola with the following parametric representation
\tag{1}k(t) = p + vt + \tfrac{1}{2}at^2
with (known) vectors p, v and a. Here p stands for the position at t = 0, v for velocity at t = 0, and a for the accelaration.
Next, there’s a line, parameterized by s,
\tag{2}l(s) = q + rs
with vectors q and r. If we restrict s to 0 ≤ s ≤ 1, then we’re looking at the line segment between q and q + r. (A nicer parametrization would be q(s-1) + rs, for the line segment between q and r, but that would make the following formulas a bit longer.)
Equating the parabola and the line, and separating for x and y coordinates, we get
\tag{3}p_x + v_xt + \tfrac{1}{2}a_xt^2= q_x +r_xs
\tag{4} p_y + v_yt + \tfrac{1}{2}a_yt^2 = q_y + r_ys
Solving (3) for s, with rx ≠ 0,
\tag{5} s = \dfrac{p_x - q_x + v_xt +\tfrac{1}{2}a_xt^2} {r_x}
Then substitute this result for s in (4). This leads to a quadratic equation,
\tfrac{1}{2}(a_y - \frac{r_y}{r_x}a_x)t^2+ (v_y-\frac{r_y}{r_x}v_x)t+p_y-q_y-\frac{r_y}{r_x}(p_x-q_x) = 0
from which one, two or zero solutions for t can be found. See below for an algorithm that will do that for you.
A solution for t means we can calculate the incidence point of the parabola and the line, using (1).
If there are solutions for t, calculate s using (5). Checking if 0 ≤ s ≤ 1 it follows whether the parabola hits the line segment between r and q + r or misses it. The incidence point(s) then follow either from (1) or (2).
We assumed above that rx ≠ 0. Otherwise (3) will lead to a quadratic equation from which t can be found.
Looking for the Bezier control point
At this stage, we have the begin and end positions (let’s call them p and q) lying on the parabola with parametrization (I repeat)
k(t) = p + vt + \tfrac{1}{2}at^2
We also have the speed vectors v and w, and we know the value of parameter t at position p and q (resp. 0 and tq).
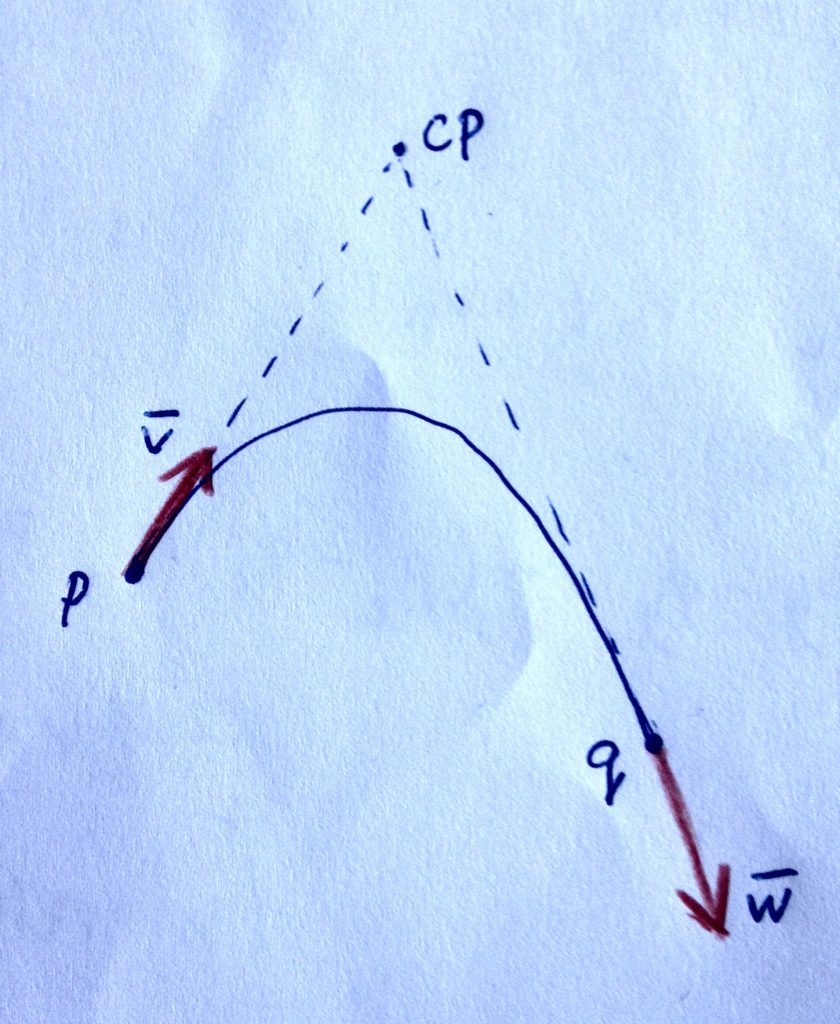
So the following formulas hold:
\tag{6} q = p + vt_q + \tfrac{1}{2}at_q^2
\tag{7} w = v + at_q
To draw the parabola from p to q on a canvas context as a quadratic Bezier curve we need to find the control point cp.
The control point is the incidence point of the line l through p with direction v, and the line m through q with direction w, see image above. We could find the incidence point of l and m with a general algorithn, however in this case there is an easier method.
The point
p + \tfrac{1}{2}t_qv
lying on l, and
q - \tfrac{1}{2}t_qw
lying on m, can be shown to coincide by substituting (6) and (7). So that must be the control point we are after.
The JavaScript code to render the graphics would look like this:
The following image shows the first bounces together with their control points (in pink).
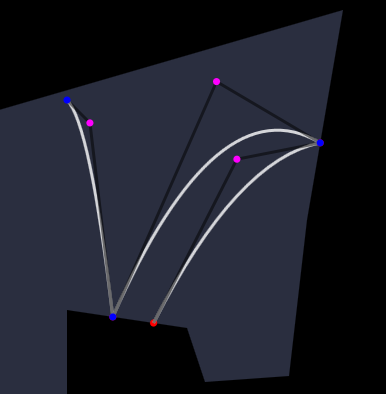
What’s left out
Apart from finding incidence points of a parabola with a line and finding control points of Bezier curves, there are a few more steps to complete the bouncing in a box example.
One thing I omittted is that to follow the trajectory of the bouncing object we need to find the first edge the parabola would hit. This means searching for the incidence point with the smallest positive t.
Another omission is calculating the new speed vector at a bouncing point.
If your interested in these steps, check the code in the github repository.